The Oracle Certified Associate (OCA) Java SE 8 exam is a crucial step for any aspiring Java developer. This exam tests your understanding of fundamental Java concepts and requires a strong grasp of various topics, In this article we will explore multiple topics including data types and variables in Java including variable declaration, instance initializers, scopes of variables, and garbage collection.
Data types in Java
Java is known as one of the most strongly typed programming languages. It has two categories of data types: primitive data types and reference data types.
Primitive Data Types In Java
Java comes with 8 built-in data types (primitive data types). These data types correspond to a fixed number of bits in memory and are used to store basic data types, such as integral numbers, characters, decimal numbers, and booleans. Here is a list of the available data types along with their memory usage.
byte
(1 byte = 8bits),short
(2 bytes = 16bits)int
(4 bytes = 32 bits),long
(8 bytes = 64bits)float
(4 bytes = 32bits),double
(8 bytes = 64bits)boolean
depends on the JVMchar
(2 bytes = 16bits)
Reference Data Types In Java
Objects and arrays are called reference types because they store references (or addresses) to the actual data, not the data itself.
Example
String str = "javalaunchpad";
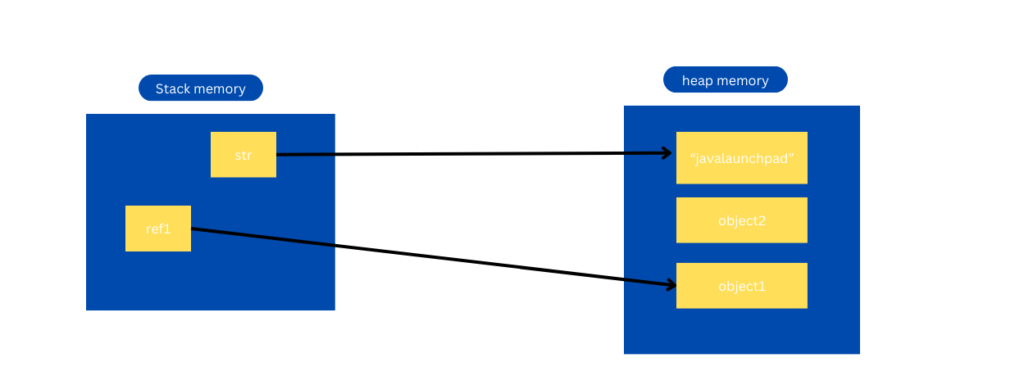
Data Types In Java- Tips & Tricks
- Decimal values are considered
double
by default; addf
to indicate afloat
(e.g.,float pi = 3.14f;
). - Underscores can be used with numeric literals for readability (e.g.,
1_000_000
), but not at the beginning, end, or adjacent to the decimal point. - Numeric literals can be represented in octal, hexadecimal, binary, and decimal formats.
- If a numeric literal exceeds the
int
size, a compilation error will occur. AddL
to treat it as along
.
Variables In Java
Variable Declaration
Variables in Java must be declared before they are used. The declaration specifies the type of the variable and optionally initializes it.
Example:
int number; // declaration
number = 10; // initialization
String message = "Hello, World!"; // declaration with initialization
Variables Initialization
Initialization is the process of assigning a value to a variable. Variables can be initialized at the time of declaration or later in the code.
Example:
public class Example {
int count = 0; // initialization at declaration
int number;
public Example() {
number = 5; // initialization in constructor
}
}
Tips & Tricks
- Identifiers (variable names) cannot be reserved keywords, start with a number, or contain characters other than
_
and$
. Starting from Java 9,_
cannot be used alone as a variable name. - It’s better to use camelCase for variable and method names, CamelCase for class/interface names, and SNAKE_CASE for constants.
- Pay attention when declaring multiple variables in the same line to avoid confusion.
- Local variables must be initialized before use, or the code will not compile.
- Instance variables (non-static fields) have default values (e.g.,
0
for integers,0.0
for floating-point numbers,false
for boolean,null
for objects,'\u0000'
for char). - Class variables (static fields) are also initialized with default values.
Instance Initializers In Java
Instance initializers are blocks of code that are executed when an instance of a class is created. They are used to initialize instance variables and are executed before the constructor. In this section, we will focus on common tricks that might appear on the exam. If you are not familiar with instance initializers, it is recommended to read an introductory article on the topic first. I recommend the following article.
Example of an Instance Initializer
public class Example {
{
System.out.println("Instance initializer block");
}
public Example() {
System.out.println("Constructor");
}
public static void main(String[] args) {
Example example = new Example();
}
}
Output:
Instance initializer block
Constructor
Common tricks
Two common tricks might come up regarding this topic in the Oracle Certified Associate Exam:
- Order of Execution
The order of execution is crucial. Fields and instance initializers are executed first, in the order they appear in the class, followed by the constructor. Exam questions might ask you to track the value of a variable whose value is changed by fields, instance initializers, and sometimes the constructor.
public class OrderExample {
int x = 10;
{
x = 20;
}
public OrderExample() {
x = 30;
System.out.println(" x = " + x);
}
public static void main(String[] args) {
OrderExample example = new OrderExample();
}
}
Output:
x = 30
- Declaration Before Use
The order in which variables are declared matters. You cannot read from a variable within an instance initializer before it is declared, though you can write to it.
Example
public class DeclarationExample {
{
System.out.println("y = " + y); // Trying to read y before it's declared will cause a compilation error
}
int y = 10;
public static void main(String[] args) {
DeclarationExample example = new DeclarationExample();
}
}
But the following code will compile successfully
public class DeclarationExample {
{
y = 400 ;
}
int y = 10;
public static void main(String[] args) {
DeclarationExample example = new DeclarationExample();
}
}
Managing Variable Scope
Variable scope defines the region of the code where a variable can be accessed. Java has several types of scopes:
- Local Scope: Variables declared inside a method or block.
- Instance Scope: Variables declared inside a class but outside any method.
- Static Scope: Variables declared with the
static
keyword inside a class.
Example:
public class ScopeExample {
static int staticVar = 10; // static scope
int instanceVar = 20; // instance scope
public void method() {
int localVar = 30; // local scope
}
}
Tips & Tricks
- A variable can only be accessed within its scope.
- Instance variables are available until the object is eligible for garbage collection.
- Class variables are available until the end of the program.
- Be cautious with shadowing, where a local variable has the same name as an instance variable. Use the
this
keyword to refer to instance variables within methods.
Garbage Collection
Garbage collection in Java is the process by which the JVM reclaims memory from objects that are no longer in use. It helps in managing memory automatically and prevents memory leaks.
Key Points:
- Automatic Memory Management: Java handles memory allocation and deallocation.
- Eligibility for GC: An object becomes eligible for garbage collection when no live thread can access it.
- Finalize Method: The
finalize
method is called by the garbage collector before the object is removed from memory, but it’s not recommended to rely on it.
Example:
public class GarbageCollectionExample {
public static void main(String[] args) {
GarbageCollectionExample obj = new GarbageCollectionExample();
obj = null; // the object is now eligible for garbage collection
System.gc(); // request for garbage collection
}
@Override
protected void finalize() throws Throwable {
System.out.println("Garbage collector called");
}
}
Tips & Tricks
- For the exam, you need to know that
System.gc()
is not guaranteed to run or do anything. - Be able to recognize when objects become eligible for garbage collection.
- Avoid unnecessary object creation to reduce the burden on the garbage collector.
- Use tools like VisualVM to monitor and analyze garbage collection in your applications.
Conclusion
Preparing for the Oracle Certified Associate Java 8 exam requires a deep understanding of various Java concepts, including data types and variables. Mastering this topic and practicing with code examples can increase your chances of passing the exam.